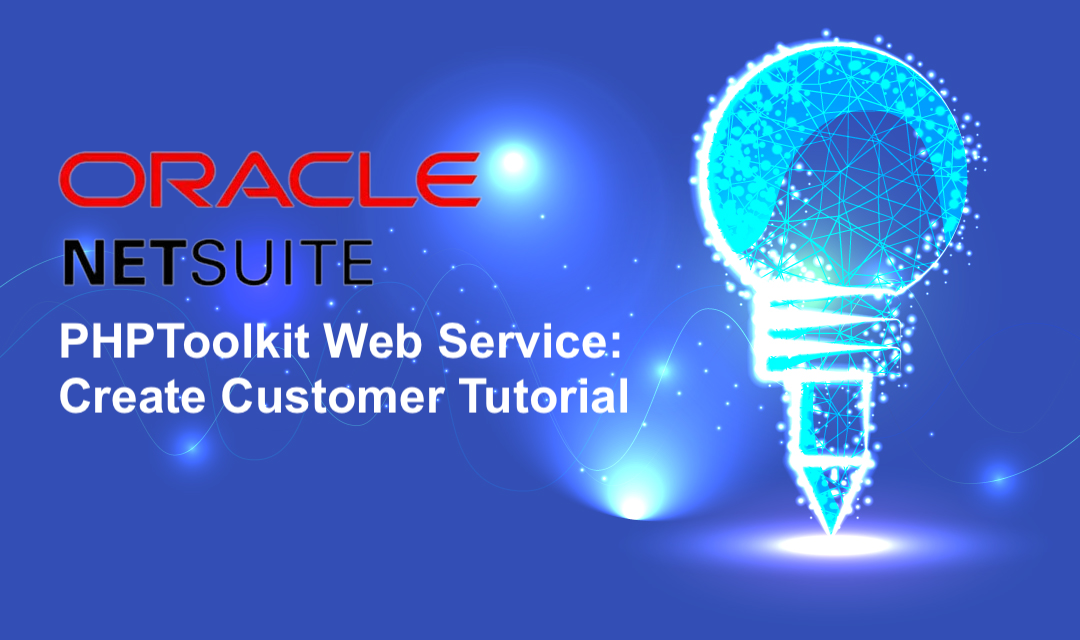
Netsuite provides a soap-based web service interface called SuiteTalk to allow 3rd-party applications to interface with the Netsuite ERP system. Netsuite also provides Toolkits written in PHP, .NET, and Java programming languages, which will make Netsuite integration easier. We'll use PHPToolkit to create a customer record in Netsuite.
In order to interface with Netsuite, we'll have to set up a login_info which we can use to authenticate in Netsuite. The PHPToolkit includes sample PHP scripts that illustrate how to add customer records (sample_add_customer.php) and search records (sample_search_items.php) in the Netsuite platform.
require ('PHPtoolkit.php'); require ('login_info.php'); class CreateCustomer { const CustomEntityTestId = 4; const CustomerEntityStatusId = 6; // LEAD::unqualified. public static function add($data) { global $myNSclient; // We are going to hard-code custom entity here for illustration purpose. $test = new nsListOrRecordRef( array('internalId' => self::CustomEntityTestId, 'typeId' => 1)); $testRef = new nsComplexObject("SelectCustomFieldRef"); $testRef->setFields(array("internalId" => 'custentity_test', 'value' => 5)); $customFieldList = new nsCustomFieldList( array("customField" => array($testRef))); // create array of customer fields $customerFields = array ( 'isPerson' => true, 'firstName' => $data['firstName'], 'lastName' => $data['lastName'], 'companyName' => $data['companyName'], 'phone' => $data['phone'], 'email' => $data['email'], 'entityStatus' => new nsRecordRef( array('internalId' => self::CustomerEntityStatusId)), 'customFieldList' => $customFieldList, ); // create Customer record $customer = new nsComplexObject('Customer'); $customer->setFields($customerFields); $response = $myNSclient->add($customer); if (!$response->isSuccess) { $response = $response->statusDetail[0]->message; } else { return TRUE; } } } $data = array("name" => "John Doe", "phone" => "333-333-3333", "email" => "[email protected]" ); $response = CreateCustomer::add($data);
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment